Creating a Cookies Bar becomes remarkably straightforward, particularly in contrast to conventional JavaScript, when incorporated into a WordPress site using jQuery. This article demonstrates the process of establishing, retrieving, and purging cookies through jQuery, offering an effortless method for WordPress users to adopt and employ.
Within this realm of options for reading, setting, and eliminating cookies, occasions may arise where the extensive feature set is excessive, prompting a preference for a streamlined yet efficient resolution.
I’ve outlined jQuery functions that I find favorable for seamlessly and promptly managing cookie-related tasks. Integrating these functions into a JavaScript file facilitates their utilization.
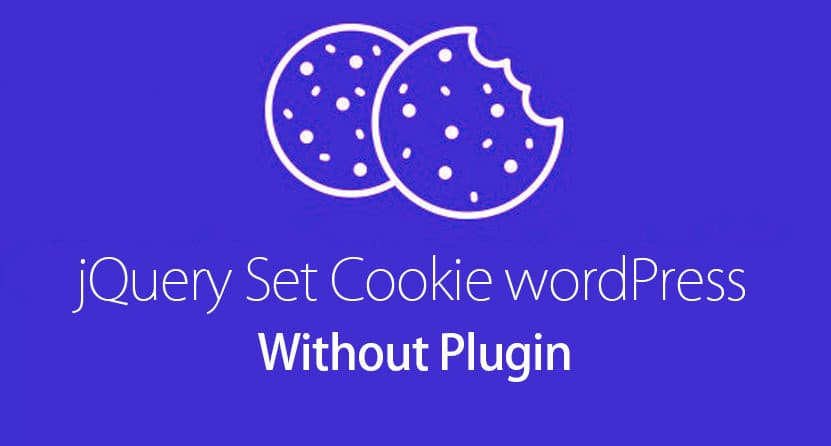
I have generated a basic trio of files: “index.html” for the webpage structure, “style.css” for styling, and “cookie.js” for JavaScript functionality.
Keep in mind that you have the option to employ jQuery cookies across various platform frameworks, including WordPress.
Step 1: Generate a file named “index.html” (For integrating with WordPress, navigate to Login → Appearance → footer.php)
Initially, an index file is required to execute and establish cookies on your website. (For WordPress users, please copy and paste the contents exclusively within the body tag.)
<!doctype html>
<head>
<meta charset="utf-8">
<title>How to set cookies with JavaScript Demo</title>
<link rel="stylesheet" type="text/css" href="style.css" />
</head>
<body>
<!-- For WordPress users copy start -->
<div id="container">
<div class="message cookie__set_message hid__sec">
<p>Displayed only the first time you visit this page. Refresh to hide it!</p>
<p>Even when you refresh the page, the browser remembers your option.</p>
</div>
<div class="message cookie__onclc hid__sec">
<p>Set Cookies hide this message, by clicking the “×” on the right of this box <a href="#?" class="close" title="Hide This Message">×</a></p>
<p>This is a new message. Even when you refresh the page, the browser remembers your option.</p>
</div>
</div>
<footer>
<div class="reset">To reset, <a href="">remove the cookies</a>.</div>
</footer>
<script type="text/javascript" src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script type="text/javascript" src="cookie.js"></script>
<script type="text/JavaScript">
$(document).ready(function() {
// COOKIES
if ($.cookie('hide-after-load') == 'yes') {
$('.cookie__set_message').removeClass('hid__sec');
$('.cookie__set_message').addClass('hide--first');
}
// if the cookie is true, hide the initial message and show the other one
if ($.cookie('hide-after-click') == 'yes') {
$('.cookie__onclc').removeClass('hid__sec');
$('.cookie__onclc').addClass('hide--first');
}
// add cookie to hide the first message after load (on refresh it will be hidden)
$.cookie('hide-after-load', 'yes', {expires: 7 });
$('.close').click(function() {
if (!$('.cookie__onclc').is('hide--first')) {
$('.cookie__onclc').removeClass('hid__sec');
$('.cookie__onclc').addClass('hide--first');
$.cookie('hide-after-click', 'yes', {expires: 7 });
}
return false;
})
//reset the cookies (not shown in tutorial)
$('.reset a').click(function() {
if (!$(this).hasClass('clicked')) {
$(this).addClass('clicked');
// add cookie setting that user has clicked
$.cookie('hide-after-load', 'no', {expires: 7 });
$.cookie('hide-after-click', 'no', {expires: 7 });
}
location.reload();
});
});
</script>
<!-- For WordPress users copy start -->
</body>
</html>
Step 2: Generate the “style.css” file (For WordPress, navigate to Appearance → style.css)
body { font-family: "Helvetica Neue", 'Helvetica', sans-serif; color: #666; margin: 1em; line-height: 2; } a:link, a:visited { color: #f00; text-decoration: none; padding-bottom: 0.1875em; } a:hover{ color: #fe580f; cursor: pointer; }
/* For WordPress Start */
.hide--first > *:first-child {
display: none;
}
.hid__sec > *:last-child {
display: none;
}
/* For WordPress End */
.message { text-align: center; width: 42%; padding: 0 2%; float: left; background: #f5deff; } .message:last-of-type { float: right; } .close { color: #f00; position: absolute; text-transform: lowercase; right: 20px; font-size: 1.5em; top: 10px; line-height: 1; border: none !important; } p.info { font-size: 19px; margin-bottom: 50px; text-align: center; } p.info span { color: #666 } footer:before{ content: ""; display: table; clear: both; } footer { padding-top: 40%; color: #888; text-align: center; }
Step 3: Create the “cookie.js” file
/*!
* jQuery Cookie Plugin v1.4.0
* https://github.com/carhartl/jquery-cookie
*
* Copyright 2013 Klaus Hartl
* Released under the MIT license
*/
(function (factory) { if (typeof define === 'function' && define.amd) { define(['jquery'], factory); } else { factory(jQuery); }}(function ($) { var pluses = /\+/g; function encode(s) { return config.raw ? s : encodeURIComponent(s); } function decode(s) { return config.raw ? s : decodeURIComponent(s); } function stringifyCookieValue(value) { return encode(config.json ? JSON.stringify(value) : String(value)); } function parseCookieValue(s) { if (s.indexOf('"') === 0) { s = s.slice(1, -1).replace(/\\"/g, '"').replace(/\\\\/g, '\\'); } try { s = decodeURIComponent(s.replace(pluses, ' ')); return config.json ? JSON.parse(s) : s; } catch(e) {} } function read(s, converter) { var value = config.raw ? s : parseCookieValue(s); return $.isFunction(converter) ? converter(value) : value; } var config = $.cookie = function (key, value, options) { if (value !== undefined && !$.isFunction(value)) { options = $.extend({}, config.defaults, options); if (typeof options.expires === 'number') { var days = options.expires, t = options.expires = new Date(); t.setTime(+t + days * 864e+5); } return (document.cookie = [ encode(key), '=', stringifyCookieValue(value), options.expires ? '; expires=' + options.expires.toUTCString() : '', options.path ? '; path=' + options.path : '', options.domain ? '; domain=' + options.domain : '', options.secure ? '; secure' : '' ].join('')); } var result = key ? undefined : {}; var cookies = document.cookie ? document.cookie.split('; ') : []; for (var i = 0, l = cookies.length; i < l; i++) { var parts = cookies[i].split('='); var name = decode(parts.shift()); var cookie = parts.join('='); if (key && key === name) { result = read(cookie, value); break; } if (!key && (cookie = read(cookie)) !== undefined) { result[name] = cookie; } } return result; }; config.defaults = {}; $.removeCookie = function (key, options) { if ($.cookie(key) === undefined) { return false; } $.cookie(key, '', $.extend({}, options, { expires: -1 })); return !$.cookie(key); };}));
Please bear in mind that you can execute this code on your local computer by running it through XAMPP or any Apache server. I trust you found this tutorial enjoyable and perhaps gathered some inspiration for your upcoming projects.